PayVault Items
PayVault includes a complete system to define, buy and manage items, that is both secure and easy to use.
Items that users can buy, we call them PayVault items, are just database objects stored in a BigDB table called PayVaultItems.
There is a method for buying items with coins: Buy(), and a method for buying items directly from PayVault providers with real-world money: GetBuyDirectInfo().
You don't have to use the items system in order to use PayVault; you can use just the coins virtual currency system if you want. Or, if you have special needs not met by the featureset of the items system, you can build your own item management system using BigDB and the Credit() and Debit() coins methods.
Defining PayVault Items
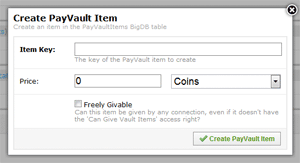
PayVault items are simply database objects stored in a BigDB table called PayVaultItems.
You can create and edit items in the PayVault Control Panel, or in any other way that BigDB objects can be edited; in the BigDB Control Panel, or through the client-side API or server-side API.
Most likely, you'll create and edit the items from the Control Panel, but it's a good idea to keep in mind that PayVault items are just regular BigDB items, and that you can create them from code if you ever need to.
Item Properties
Since PayVault items are just BigDB database objects, you can add as many properties as you want when defining your items.
For instance, if you were building a racing game, you might create a 'truck' item like so:
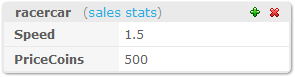
Setting a price in Coins
If you want users to be able to buy an item with coins from their vault, you'll need to set a price in coins, which is done simply by adding a 'PriceCoins' property to the item. The 'PriceCoins' property must have an positive integer value such as 2 or 5000. Example:
Setting a price in real-world currency
Some PayVault providers, but not all, support buying items directly with real-world currency which completely bypasses the coins virtual currency system.
This feature is useful when you're just selling one or two items, such as the full version of your game, and don't want to introduce an entire virtual currency system to your users.
If you want an item to be buyable with using these direct purchases, you'll need to define a price of the item in one or more real-world currencies so the PayVault providers knows what to charge for the item.
Real-world currency prices should be integer properties and have names like 'PriceUSD' or 'PriceYEN', and the value should be the price in minor units, e.g. cents. For example:
Downloading vault contents
The user's vaults contains their coin balance and any items they might have. Before you can access either of those from your client code, you'll have to update the contents of the user's vault using one of these methods:
-
Call Refresh()
Whenever you call Refresh(), the entire contents of the users vault will be downloaded from the PayVault Backend Service.
Once the vault contents has been downloaded once by any method, Refresh() will cause the system to only send the vault contents if the contents have changed since they were last downloaded, in order to save bandwidth. -
Call any of the methods that modify the vault contents
The complete contents of a users vault, including the coin balance, is always downloaded after all the methods calls that modify the vault contents, such as Credit(), Debit(), Buy() and Consume(). This is done so you don't have to call Refresh() after every other method call.
Listing items
Items that users have bought or been given are stored in their vaults.
You can always access the current items in a users vault via the Items property of the PayVault class, but note that you have to have downloaded the contents of the vault as described above before you attempt to read the property, or you'll get an error.
When you retrieve the list of items in a user's vault, you will get back VaultItem objects which are very similar to BigDB objects, except that they're read-only and they contain these purchase-related properites:
- Id: Each vault item is given a unique id, so you can distinguish between multiple items of the same type (ItemKey).
- PurchaseData: When the item was added to the vault.
- ItemKey: The key of the underlying PayVault item from the PayVaultItems table.
Each VaultItem also has all the properties from the underlying PayVaultItem, possibly combined with the properties from a payload (see below for information about payload).
Here is an example of listing all items currently in the vault:
Convenience methods: Has(), First() and Count()
Althought you could get the same information simply by looping over the items property, we provide a few simple convenience methods that makes it easy to examine the items in a users vault
- Has(itemKey): Returns true if the user has at least one item of the given type (itemKey).
- First(itemKey): The first item of the given type (itemKey), or null if the user doesn't have any such item.
- Count(itemKey): How many items of the given type (itemKey) the user currently has.
Buying items with coins: Buy()
Buying an item with coins is incredibly straight-forward. Simply call the Buy method with the items that the user wants to purchase. If the user has enough coins to afford the items, the transaction goes through, and the vault is updated with the new coin balance and the new items.
Payloads
If the user buys the 'racercar' item:
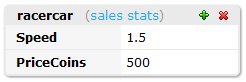
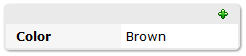
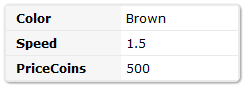
When buying or giving items, you can optionally specify a payload as an easy way to customize the items.
For instance, instead of having 10 different PayVault items for cars in 10 colors, you might you might have a single 'car' item with all the properties of a car such as 'speed','acceleration','topspeed' and use payload to specify the color of the car at purchase time.
Payloads are merged with the underlying PayVault item when listing vault items. However, properties of the underlying PayVault item always takes precedence over properties with the same name in the payload. So if the PayVault item defines a 'speed' property, you can't override it using payload. This is a security measure to prevent users from buying a cheap item and using payload to modify it into a more expensive item.
Payloads can't be changed with code, but the Control Panel does allow you to manually edit payloads of vault items.
Payloads are meant for minor customizations of PayVault items, and you shouldn't be putting much more than a few properties into a payload.
As an example, here the user buys the 'truck' item defined earlier, but uses payload to define the size of the truck.
Giving items to users: Give()
It is also possible to call the Give() method to simply give items to users without any payment. This can be used to give out the occasional bonus item to users.
By default, only trusted serverside code can call this method. To be able to give any item from your clientside code, you can add the right to the connection your clientside code is using in the Connections section of the Control Panel.
Give() example:
Freely giveable items
Sometimes you want to be able to give some items, like insignificant powerups, from clientside code without giving the clientside code rights to give any item at any time.
To facilitate this, you can mark an item as Freely Givable, which means that access rights won't be checked when trying to use Give() to give the item. This is done simply by adding a Boolean property called 'FreelyGivable' to the item with the value true. Example:
Consuming items from vault: Consume()
To remove an item from a user's vault, you can call the Consume() method. You can consume a single or multiple items at the same time.
Note that PayVault doesn't support buying and consuming counts of items directly. It is however possible to buy several items with the same itemkey and the same extra properties at the same time, and it is also possible to consume several of those items at the same time. But in the item list, each item is unique.
Buying items with real-world currency: GetBuyDirectInfo()
It is also possible to let users buy items directly, without using Coins, by calling the the GetBuyDirectInfo() method with a provider and the required purchase arguments.
You'll get back everything you need to initiate payment for the user, such as a popup URL for PayPal or the parameters for a Kongregate API call.
Since the purchase arguments are provider specific, you should go to the PayVault Provider Documentation to find the various purchase arguments accepted by each provider.
You will have to implement a system to know when the payment is complete. For example, if you overlayed an iframe over the game or open a popup, you can use javascript to figure out when the user closes the payment flow. Or, if you have no idea of knowing when the user finishes the payment flow, you can display a 'waiting for payment' dialog in-game, and call Refresh() every second or so while the dialog is shown. It all depends on what environment your game runs in, and which provider you're using.
To be able to purchase items directly, the items have to have a price in an actual currency. To directly buy one of the cars as defined above, you could do this:
Item Bundles
Sometimes you might want to sell bundles of items at a discounted price. Normally, if a user buys multiple items at once, PayVault will sum up the price of those items and charge that, but with bundles it is possible to define special PayVault items that when bought are exchanged for the actual items.
For example, say that in your racing game you sell better wheels, better engines and better brakes for players' cars, and that you want to offer a discount to players who buy all three at once. By setting up a Bundle item you can do just that. In the PayVault Item Control Panel, simply add a property to your item called 'Bundle', make it an array, and add the keys of the items you want it to contain.
In this example, when a user buys the item 'carpack', he will be charged its price, but when the purchase is successful, his Vault won't contain the 'carpack' item, but instead one each of the 'betterwheels', 'betterengine', and 'betterbrakes' items.
It's of course possible to include multiples of the same item, simply add it multiple times to the 'Bundle' array. For example, if you normally sell an item called 'speedboost' for 100 coins, if you define an item like this, you can sell 5 of those for the discounted price of 400 coins:
User Experience
Just like the other Backend Services, you're 100% in control of the user experience. For instance, there is no built-in graphical shop interface provided by PlayerIO. If you want to present a shop to your users you have to build it yourself, but this gives you the advantage of having a shop system tailored directly to your game.
Since PayVault Items are just database objects in a BigDB table, you can use the regular BigDB API methods to list items to display in your shop. You should also feel free to add additional indexes to the PayVaultItems table, if your game need to list items in any game specific order.